Overview
Learn how we manage customers in Bloc.
Customers are the foundation of every product in Bloc. We use customers to manage your users and connect them to your organization. When exploring the Bloc API, especially with issuing APIs (virtual accounts and cards), you will notice that you cannot issue a virtual account or card to a customer that doesn't exist in your account.
The first and most important step to integrating our banking as a service API is to create a customer. Everything else is layered on top of this.
Get all customers
To get all of the customers in your organisation, call the endpoint URL: https://api.blochq.io/v1/customers
.
const sdk = require('api')('@bloc/v1.0#1lj3mr4welh6dgcg7');
sdk.auth('{secretKey}');
sdk.getcustomers()
.then(({ data }) => console.log(data))
.catch(err => console.error(err));
require 'uri'
require 'net/http'
require 'openssl'
url = URI("https://api.blochq.io/v1/customers")
http = Net::HTTP.new(url.host, url.port)
http.use_ssl = true
request = Net::HTTP::Get.new(url)
request["accept"] = 'application/json'
request["authorization"] = 'Bearer {secretKey}'
response = http.request(request)
puts response.read_body
<?php
require_once('vendor/autoload.php');
$client = new \GuzzleHttp\Client();
$response = $client->request('GET', 'https://api.blochq.io/v1/customers', [
'headers' => [
'accept' => 'application/json',
'authorization' => 'Bearer {secretKey}',
],
]);
echo $response->getBody();
import requests
url = "https://api.blochq.io/v1/customers"
headers = {
"accept": "application/json",
"authorization": "Bearer {secretKey}"
}
response = requests.get(url, headers=headers)
print(response.text)
Get Customer by ID
All customers created via the Bloc API have a customer_id
. To get the details of a customer, you need to call the endpoint URL below, and provide the id
of the customer in {customerID} placeholder.
const sdk = require('api')('@bloc/v1.0#1lj3mr4welh6dgcg7');
sdk.auth('{secretKey}');
sdk.getcustomerbyid({customerID: '{customerID}'})
.then(({ data }) => console.log(data))
.catch(err => console.error(err));
require 'uri'
require 'net/http'
require 'openssl'
url = URI("https://api.blochq.io/v1/customers/{customerID}")
http = Net::HTTP.new(url.host, url.port)
http.use_ssl = true
request = Net::HTTP::Get.new(url)
request["accept"] = 'application/json'
request["authorization"] = 'Bearer {secretKey}'
response = http.request(request)
puts response.read_body
<?php
require_once('vendor/autoload.php');
$client = new \GuzzleHttp\Client();
$response = $client->request('GET', 'https://api.blochq.io/v1/customers/{customerID}', [
'headers' => [
'accept' => 'application/json',
'authorization' => 'Bearer {secretKey}',
],
]);
echo $response->getBody();
import requests
url = "https://api.blochq.io/v1/customers/{customerID}"
headers = {
"accept": "application/json",
"authorization": "Bearer {secretKey}"
}
response = requests.get(url, headers=headers)
print(response.text)
Here's the response you get
{
"success": true,
"data": {
"id": "{customerID}",
"full_name": "Oladeji Ayo",
"phone_number": "08012345678",
"organization_id": "{organizationID}",
"environment": "live",
"email": "ayooladeji@abc.xyz",
"country": "",
"group": "main",
"status": "active",
"created_at": "2023-03-08T10:59:39.509Z",
"updated_at": "2023-03-17T12:59:13.403Z",
"first_name": "Ayo ",
"last_name": "Oladeji",
"kyc_tier": "1",
"bvn": "00000000000",
"place_of_birth": "Kaduna",
"gender": "male",
"image_url": "{imageURL}",
"date_of_birth": "2001-04-14T00:00:00Z",
"customer_type": "Personal",
"source": "Banking",
"address": {
"street": ""
}
},
"message": "customer"
}
Please note
- {customerID}, {imageURL}, {organizationID}, and {secretKey} shown in the sample requests and response above are placeholders.
Attributes
Name | Type | Description |
---|---|---|
success | string | Shows the status of your API request. |
data | object | Contains all of the information regarding the particular customer whose details you tried to get. |
id | string | The assigned ID of the customer |
full_name | string | Full name of the customer (surname first) |
phone_number | string | Phone number of the customer |
organization_id | string | Your organization ID |
environment | string | The environment in which the customer was created in — Live or Test Mode. |
string | Email address of the customer | |
country | string | Country of residence of the customer |
status | string | Status of the customer. This can be active or archived |
created_at | string | Time the customer was created |
updated_at | string | The last time the customer details were updated. |
first_name | string | First name of the customer |
last_name | string | Last name of the customer |
kyc_tier | string | The KYC tier of the customer |
bvn | string | The Bank Verification Number of the customer |
place_of_birth | string | Place of birth of the customer |
gender | string | Gender of the customer |
image_url | string | The image address of the customer's passport photo. This can be a URL or a base64 string. |
date_of_birth | string | Date of birth of the customer |
customer_type | string | Type of customer. It could be personal or business. We only allow personal at the moment. |
source | string | How the customer was created. |
address | object | Address of residence of the customer |
street | string | Street of residence of the customer |
city | string | City of residence of the customer |
state | string | State of residence of the customer |
message | string | Response message from Bloc's system |
Important to Note:
You cannot delete a customer on Bloc once they've been created. You can make them archived or edit their details. But once a customer is added, you cannot remove them.
On the Bloc Dashboard
On the Dashboard, you can view the full details of a customer by clicking on View Customer Details from the Customers page. On the Details page, you can view the total number of transactions performed by a customer, the number of debit and credit transactions, a list of their transaction activity, and a list of all the accounts & cards that have been issued to the customer.
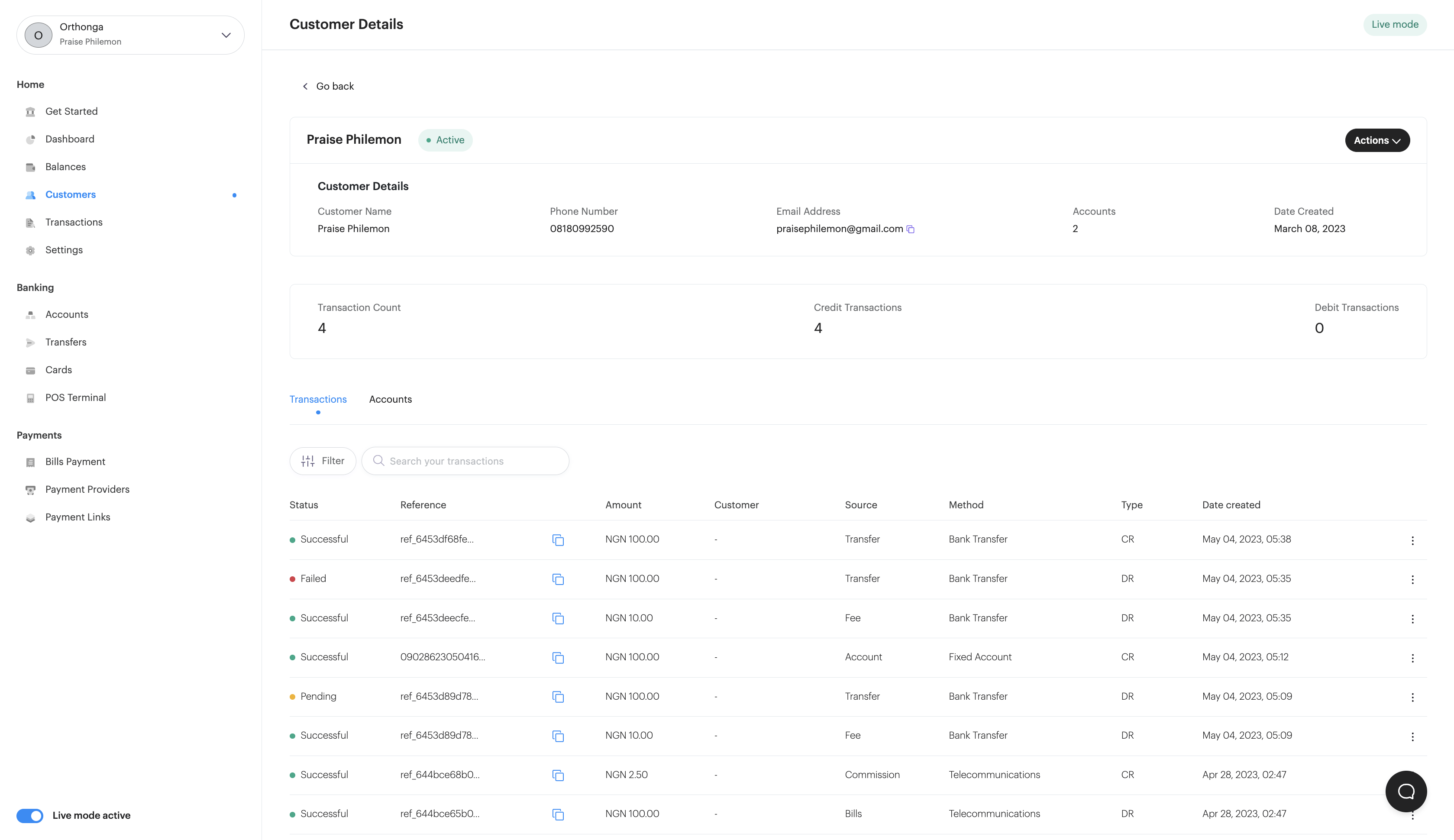
Updated almost 2 years ago