How Checkout Works
Checkout widget
Bloc Checkout widget is an easy-to-use client-side integration that provides your customers with multiple payment options.
Step 1: Call checkout endpoint
https://api.blochq.io/v1/checkout/new
var myHeaders = new Headers();
myHeaders.append("Authorization", "Bearer <YOUR-PUBLIC-KEY>");
myHeaders.append("Content-Type", "application/json");
var raw = JSON.stringify({
"customer_email": "[email protected]",
"customer_name": "hello",
"currency": "NGN",
"amount": 100000,
"reference": "my-ref"
});
var requestOptions = {
method: 'POST',
headers: myHeaders,
body: raw,
redirect: 'follow'
};
fetch("https://api.blochq.io/v1/checkout/new", requestOptions)
.then(response => response.text())
.then(result => console.log(result))
.catch(error => console.log('error', error));
<?php
require_once 'HTTP/Request2.php';
$request = new HTTP_Request2();
$request->setUrl('https://api.orchestrate.finance/v1/checkout/new');
$request->setMethod(HTTP_Request2::METHOD_POST);
$request->setConfig(array(
'follow_redirects' => TRUE
));
$request->setHeader(array(
'Authorization' => 'Bearer <YOUR-PUBLIC-KEY>',
'Content-Type' => 'application/json'
));
$request->setBody('{\n "customer_email": "[email protected]",\n "customer_name": "hello",\n "country": "Nigeria",\n "amount": 100000,\n "reference": "my-ref"\n}');
try {
$response = $request->send();
if ($response->getStatus() == 200) {
echo $response->getBody();
}
else {
echo 'Unexpected HTTP status: ' . $response->getStatus() . ' ' .
$response->getReasonPhrase();
}
}
catch(HTTP_Request2_Exception $e) {
echo 'Error: ' . $e->getMessage();
}
curl --location --request POST 'https://api.orchestrate.finance/v1/checkout/new' \
--header 'Authorization: Bearer <YOUR-PUBLIC-KEY>' \
--header 'Content-Type: application/json' \
--data-raw '{
"customer_email": "[email protected]",
"customer_name": "hello",
"country": "Nigeria",
"amount": 100000,
"reference": "my-ref"
}'
package main
import (
"fmt"
"strings"
"net/http"
"io/ioutil"
)
func main() {
url := "https://api.orchestrate.finance/v1/checkout/new"
method := "POST"
payload := strings.NewReader(`{
"customer_email": "[email protected]",
"customer_name": "hello",
"country": "Nigeria",
"amount": 100000,
"reference": "my-ref"
}`)
client := &http.Client {
}
req, err := http.NewRequest(method, url, payload)
if err != nil {
fmt.Println(err)
return
}
req.Header.Add("Authorization", "Bearer <YOUR-PUBLIC-KEY>")
req.Header.Add("Content-Type", "application/json")
res, err := client.Do(req)
if err != nil {
fmt.Println(err)
return
}
defer res.Body.Close()
body, err := ioutil.ReadAll(res.Body)
if err != nil {
fmt.Println(err)
return
}
fmt.Println(string(body))
}
Step 2: Get the Checkout URL from the response
Calling the checkout API returns a checkout URL
{
"success": true,
"data": "https://checkout.blochq.io/checkout?id=62b55e1cdb4f39116566d2bf",
"message": "checkout created"
}
Step 3: Open the checkout URL in a new tab or iframe
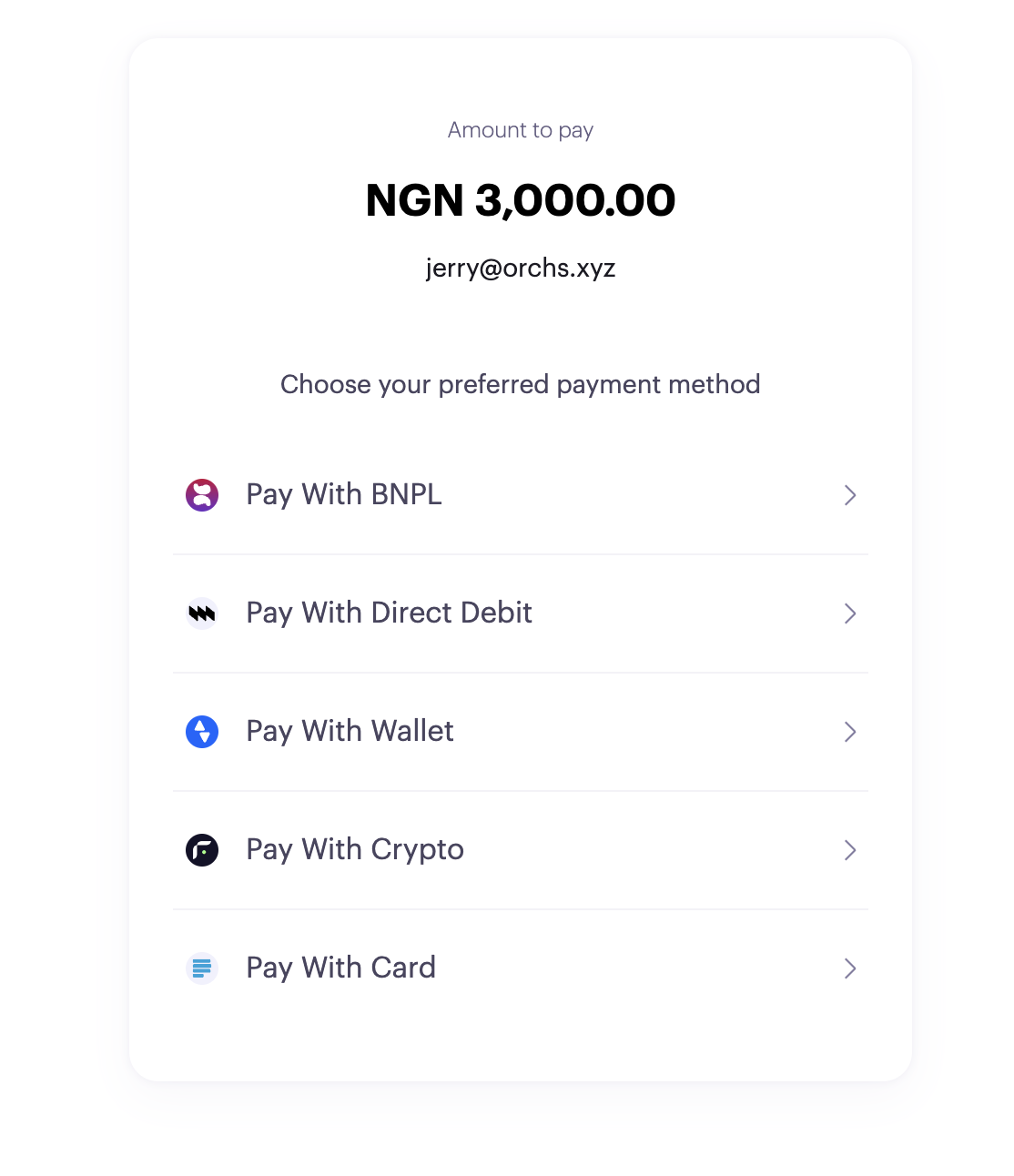
Step 4: Set up Webhook to get payment events.Webhooks
We send payment events to your webhook URL after payment has been completed.
Config Parameters
{
"customer_email": "[email protected]",
"customer_name": "akin lee",
"country": "United States",
"items": [
{
"name": "iphone",
"amount": 100000,
"quantity": 1
}
],
"amount": 100000,
"reference": "my-ref-2",
"redirect_url": "https://myapp.com/success",
"close_url": "https://myapp.com"
}
Parameter | Type | Description | Required |
---|---|---|---|
public_key | string | Your public key is how you authorize your request. Your public key can be found on your Bloc Dashboard | True |
customer_email | string | Email address of the customer. | True |
customer_name | string | Name of the customer | False |
currency | string | Transaction currency | True |
amount | number | The amount should be passed in its lowest denominator. e.g 1000 Kobo. | True |
close_url | string | When the checkout is closed we redirect the customer to the close_url provided. | False |
redirect_url | string | When a payment is completed we redirect the customer to the redirect_url provided. if none is provided we turn the customer to a generic successful page. | False |
reference | string | A unique string to identify your payment. If none is passed we auto generate a reference. | False |
Updated over 1 year ago